동구의_C# & Unity_개발일지
2024.01.02 내일배움캠프 7일차 TIL C#_ProGramming_2 본문
학습법 특강을 진행하였다.
어떤 개발자가 되어야 하는지 어떤 개발자가 되면 좋은지 공부는 어떻게 해야 되는지 등을 알려주셨다.
학습법 특강
Not Coder
Be Developer
어떻게 하면 진짜 개발자가 될 수 있을까?
1. 습관화 나의 자산을 만들기 - TIL, WIL, 알고리즘
2. 내용 내용 복습하며 사고력과 구현력 중점적으로 단련 - 개인 과제, 팀 과제
3. 기술적 의사결정 기술적 고민을 동시에 - 프로젝트
4. 메타인지, 수료 때까지 도달해야하는 실력 목표 - 개발역량점검표
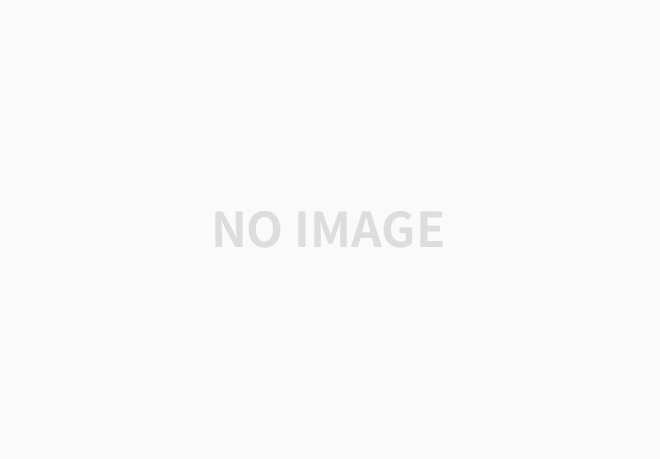
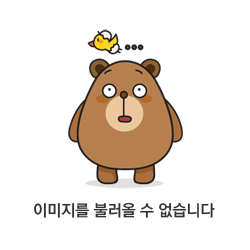
성장 가능성
다른 사람과 협업
함께 성장
끊임없는 고민
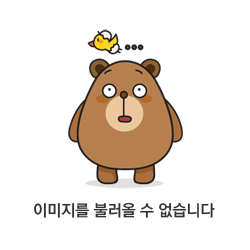
C#_ProGramming_chapter2: 과제
2-1 구구단 출력하기
1부터 9까지의 숫자를 각각 1부터 9까지 곱한 결과를 출력하는 프로그램을 작성해보세요.
namespace ConsoleApp2
{
internal class Chapter2
{
static void Main(string[] args)
{
for (int i = 2; i <= 9; i++)
{
for (int j = 1; j <= 9; j++)
{
Console.WriteLine(i + " x " + j + " = " + (i * j));
}
}
}
}
}
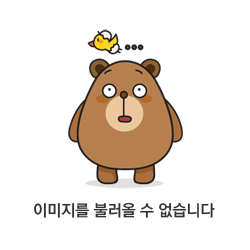
2-2 별 찍기
1. 오른쪽으로 기울어진 직각삼각형 출력하기:
이 부분에서는 for 루프를 사용하여 오른쪽으로 기울어진 직각삼각형을 출력하는 프로그램을 작성해야 합니다. 삼각형의 높이는 5입니다.
2. 역직각삼각형 출력하기:
역직각삼각형을 출력하는 프로그램을 작성해야 합니다. 이 삼각형의 높이 역시 5입니다.
3. 피라미드 출력하기: 피라미드를 출력하는 프로그램을 작성해야 합니다. 이 피라미드의 높이는 5입니다.
namespace ConsoleApp2
{
internal class Chapter2
{
static void Main()
{
for (int i = 1; i <= 5; i++)
{
for (int j = 1; j <= i; j++)
Console.Write("*");
Console.WriteLine();
}
Console.WriteLine("\n");
for (int i = 5; i >= 1; i--)
{
for (int j = 1; j <= i; j++)
Console.Write("*");
Console.WriteLine();
}
for (int i = 1; i <= 5; i++)
{
for (int j = 1; j <= 5 - i; j++)
Console.Write(" ");
for (int j = 1; j <= 2 * i - 1; j++)
Console.Write("*");
Console.WriteLine();
}
}
}
}

2-3 최대값, 최소값 찾기
사용자로부터 일련의 숫자를 입력받아, 그 중에서 최대값과 최소값을 찾는 프로그램을 작성해보세요.
// 내가 작성한 코드
namespace ConsoleApp2
{
internal class Chapter2
{
static void Main()
{
int max = int.MinValue;
int min = int.MaxValue;
for (int i = 0; i < 5; i++)
{
Console.Write("숫자를 입력하세요: ");
int userInput = int.Parse(Console.ReadLine());
if (userInput > max)
{
max = userInput;
}
if (userInput < min)
{
min = userInput;
}
}
Console.WriteLine("최대값: " + max);
Console.WriteLine("최소값: " + min);
}
}
}
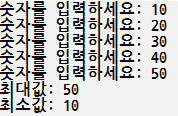
// 풀이 코드
using System;
class Program
{
static void Main()
{
int[] numbers = new int[5]; // 5개의 정수를 저장할 배열 선언
for(int i = 0; i < 5; i++)
{
Console.Write("숫자를 입력하세요: "); // 사용자에게 숫자 입력 요청
numbers[i] = int.Parse(Console.ReadLine()); // 사용자가 입력한 값을 배열에 저장
}
int max = numbers[0]; // 최대값을 배열의 첫 번째 요소로 초기화
int min = numbers[0]; // 최소값을 배열의 첫 번째 요소로 초기화
for(int i = 1; i < 5; i++)
{
if(numbers[i] > max) // 현재 요소가 최대값보다 크다면
{
max = numbers[i]; // 현재 요소를 새로운 최대값으로 설정
}
if(numbers[i] < min) // 현재 요소가 최소값보다 작다면
{
min = numbers[i]; // 현재 요소를 새로운 최소값으로 설정
}
}
Console.WriteLine("최대값: " + max); // 계산된 최대값 출력
Console.WriteLine("최소값: " + min); // 계산된 최소값 출력
}
}
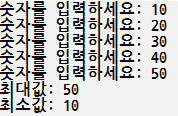
풀이에서 사용된 코드는 배열을 사용한 코드이다.
배열을 사용하여도 구할 수 있다는 것을 알았다!!
2-4 소수 판별하기
사용자로부터 숫자를 입력받아 그 숫자가 소수인지 아닌지를 판별하는 프로그램을 작성해보세요.
namespace ConsoleApp2
{
internal class Chapter2
{
static bool IsPrime(int num)
{
if (num <= 1)
{
return false;
}
for (int i = 2; i * i <= num; i++)
{
if (num % i == 0)
{
return false;
}
}
return true;
}
static void Main()
{
Console.Write("숫자를 입력하세요: ");
int num = int.Parse(Console.ReadLine());
if (IsPrime(num))
{
Console.WriteLine(num + "은 소수입니다.");
}
else
{
Console.WriteLine(num + "은 소수가 아닙니다.");
}
}
}
}


2-5 숫자 맞추기
컴퓨터는 1에서 100까지의 숫자를 임의로 선택하고, 사용자는 이 숫자를 맞추어야 합니다. 사용자가 입력한 숫자가 컴퓨터의 숫자보다 크면 "너무 큽니다!"라고 알려주고, 작으면 "너무 작습니다!"라고 알려줍니다. 사용자가 숫자를 맞추면 게임이 종료됩니다.
namespace ConsoleApp2
{
internal class Chapter2
{
static void Main(string[] args)
{
int guess = 0;
int count = 0;
Random random = new Random();
int numberToGuess = random.Next(1, 101);
Console.WriteLine("숫자 맞추기 게임을 시작합니다. 1에서 100까지의 숫자 중 하나를 맞춰보세요.");
while(guess != numberToGuess)
{
Console.Write("숫자를 입력하세요: ");
guess = int.Parse(Console.ReadLine());
count++;
if(guess < numberToGuess)
{
Console.WriteLine("너무 작습니다!");
}
else if(guess > numberToGuess)
{
Console.WriteLine("너무 큽니다!");
}
else
{
Console.WriteLine("축하합니다! " + count + "번 만에 숫자를 맞추셨습니다!", count);
}
}
}
}
}
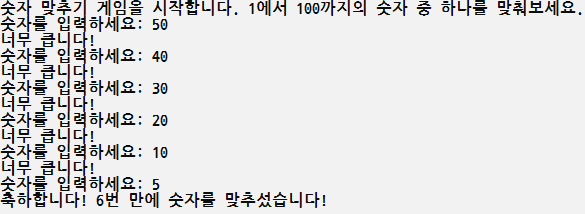
2-6 틱택토 ( 콘솔 )
namespace ConsoleApp2
{
internal class Chapter2
{
static char[,] array2 = new char[3, 3]
{
{'1', '2', '3' },
{'4', '5', '6'},
{'7', '8', '9'}
};
static void game()
{
Console.WriteLine("플레이어 1: X 와 플레이어 2: O ");
Console.WriteLine();
Console.WriteLine(" {0} | {1} | {2}", array2[0, 0], array2[0, 1], array2[0, 2]);
Console.WriteLine("---|---|---");
Console.WriteLine(" {0} | {1} | {2}", array2[1, 0], array2[1, 1], array2[1, 2]);
Console.WriteLine("---|---|---");
Console.WriteLine(" {0} | {1} | {2}", array2[2, 0], array2[2, 1], array2[2, 2]);
Console.WriteLine();
}
static void Main(string[] args)
{
int userInput;
int count = 1;
while (true)
{
Console.Clear();
game();
if (count % 2 == 0)
{
Console.WriteLine("플레이어 2의 차례");
}
else
{
Console.WriteLine("플레이어 1의 차례");
}
userInput = int.Parse(Console.ReadLine()) - 1;
int row = userInput / 3;
int column = userInput % 3;
if (array2[row, column] == 'x' || array2[row, column] == '0')
{
continue;
}
if (count % 2 == 0)
{
array2[row, column] = '0';
}
else
{
array2[row, column] = 'x';
}
count++;
if (array2[row, 0] == array2[row, 1] && array2[row, 1] == array2[row, 2])
{
Console.Clear();
Console.WriteLine("플레이어 {0}의 승리입니다!", (count % 2) + 1);
break;
}
else if (array2[0, column] == array2[1, column] && array2[1, column] == array2[2, column])
{
Console.Clear();
Console.WriteLine("플레이어 {0}의 승리입니다!", (count % 2) + 1);
break;
}
else if (array2[0, 0] == array2[1, 1] && array2[1, 1] == array2[2, 2] || array2[0, 2] == array2[1, 1] && array2[1, 1] == array2[2, 0])
{
Console.Clear();
Console.WriteLine("플레이어 {0}의 승리입니다!", (count % 2) + 1);
break;
}
if (count > 9)
{
Console.Clear();
Console.WriteLine("무승부 입니다!");
Console.WriteLine();
break;
}
}
game();
}
}
}
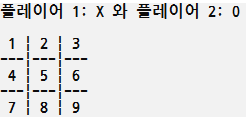

틱택토라는 게임을 만드는 과정에서 중간중간에 막혀 이것저것 많이 참고 하였다.
(사실 개인 과제 할 생각에 마음이 급해...ㅠㅠ)
그러다 보니 내가 만든 게 맞나....?
이번 2주차 마지막 과제인 틱택토 게임은 추후에 코드를 한줄한줄 분석해 고민하고 생각하면서 이해하는 시간을 가져야겠다.
'C#' 카테고리의 다른 글
2024.01.04 내일배움캠프 9일차 TIL C#_ProGramming(C# 문법) (0) | 2024.01.04 |
---|---|
2024.01.03 내일배움캠프 8일차 TIL C#_ProGramming_2(C# 문법) (0) | 2024.01.04 |
2024.01.03 내일배움캠프 8일차 TIL C#_ProGramming(Text_Game) (0) | 2024.01.03 |
2024.01.02 내일배움캠프 7일차 TIL C#_ProGramming(C# 문법) (1) | 2024.01.02 |
2023.12.29 내일배움캠프 6일차 TIL C#_ProGramming(C# 문법) (0) | 2023.12.29 |